How to securely serialize and unserialize data in Magento 2?
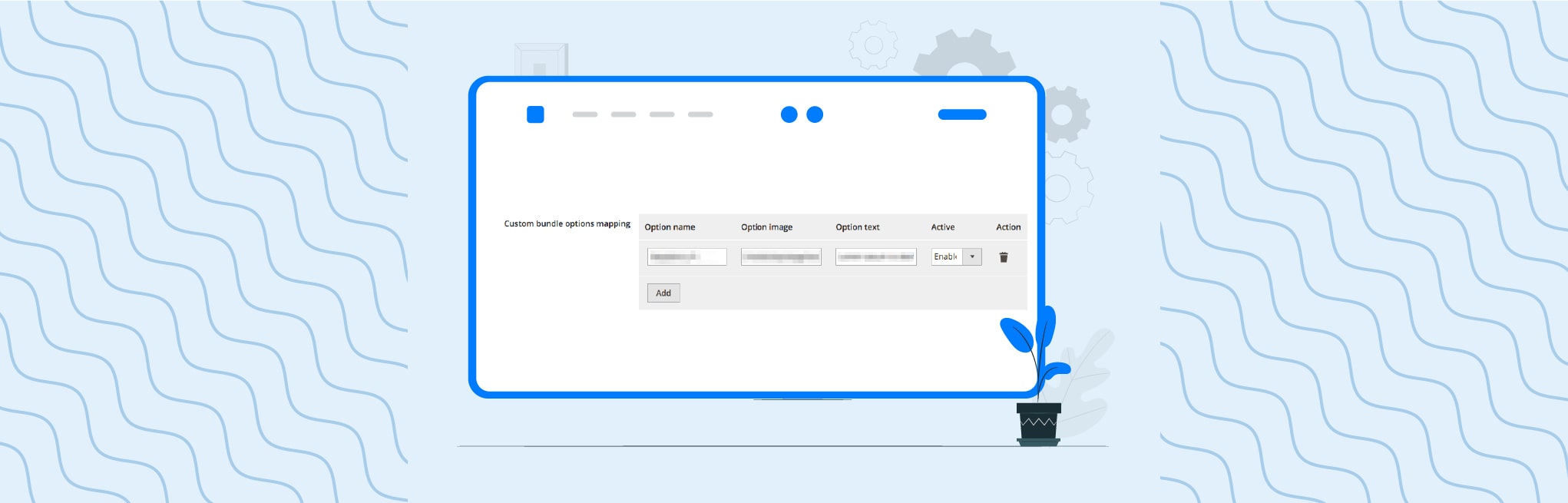
In the article, we will be going to see how data can be securely serialized and unserialized in Magento 2.
The new Magento 2.2 release replaces the usage of default PHP serialized format to JSON format. This library provides a secure way of serializing and unserializing strings, integers, floats, booleans, and arrays.
Magento’s Serialize library provides the JSON and Serialize implementations for serializing data.
Serialization.
Serialization in Magento 2 allows converting data into a string using serialize() method to store in a database, a cache, or pass onto another layer in the application.
The serialize method is used for storing or passing PHP values without losing their data type and structure.
The other half of this process uses the unserialize() function to reverse the process and convert a serialized string back into a string, integer, float, boolean, or array data.
Use the Magento\Framework\Serialize\SerializerInterface interface to create serialize data using Magento 2.
For security reasons, SerializerInterface implementations, such as the JSON and Serialize classes, should not serialize and unserialize objects.
How to Implement it.
By the following way, anyone can implement serialization in Magento 2.
JSON (default)
Magento\Framework\Serialize\Serializer\Json class is used to serializes and unserializes data using the JSON format, Please note this class did not unserialize objects
Serialize
Magento\Framework\Serialize\Serializer\Serialize class is less secure than the JSON implementation but provides better performance on large arrays. This class does not unserialize objects in PHP 7.
Magento discourages using the Serialize implementation directly because it can lead to security vulnerabilities. Always use the SerializerInterface for serializing and unserializing.
Now, we’re going to save simple custom data inside the cache and load it when Magento renders the template using the serializerInterface.
Prerequisites
First of all, you will need a Magento 2 installation which is currently 2.2 version or higher. Because this implementation of the interface that we will be using for serializing data is not available in versions bellow 2.2.
Please follow the below steps to implement the solution.
Step 1:Create a custom module under the app/code directory with two require file registration.php and etc/module.xml.
File Path: <root>/app/code/Aureatelabs/SerializerDemo/registration.php
- Where Aureatelabs is a vendor name and SerializerDemo is a module name.
<?php
/**
* SerializerDemo
*
* @category SerializerDemo
* @package Aureatelabs_DemoModule
* @license http://www.gnu.org/licenses/gpl-3.0.html
*/
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Aureatelabs_SerializerDemo',
__DIR__
);
File Path: <root>/app/code/Aureatelabs/SerializerDemo/etc/module.xml
<?xml version="1.0" ?>
<!--
/**
* SerializerDemo
*
* @category SerializerDemo
* @package Aureatelabs_SerializerDemo
* @license http://www.gnu.org/licenses/gpl-3.0.html
*/
-->
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Aureatelabs_SerializerDemo" setup_version="1.0.0"/>
</config>
Step 2: Create routes XML file inside the etc directory.
File Path: <root>/app/code/Aureatelabs/SerializerDemo/etc/frontend/routes.xml
<?xml version="1.0" ?>
<!--
/**
* SerializerDemo
*
* @category SerializerDemo
* @package Aureatelabs_SerializerDemo
* @license http://www.gnu.org/licenses/gpl-3.0.html
*/
-->
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="../../../../../../lib/internal/Magento/Framework/App/etc/routes.xsd">
<router id="standard">
<route id="serializerdemo" frontName="serializerdemo">
<module name="Aureatelabs_SerializerDemo" />
</route>
</router>
</config>
Step 3: Create a controller class inside the controller directory.
File Path: <root>/app/code/Aureatelabs/SerializerDemo/Controller/Index/Index.php
<?php
namespace Aureatelabs\SerializerDemo\Controller\Index;
class Index extends \Magento\Framework\App\Action\Action
{
/**
* @return void
*/
public function execute()
{
$this->_view->loadLayout();
$this->_view->getLayout()->initMessages();
$this->_view->renderLayout();
}
}
Step 4: Create a block class inside the block directory.
File Path: <root>/app/code/Aureatelabs/SerializerDemo/Block/Index/Index.php
<?php
namespace Aureatelabs\SerializerDemo\Block\Index;
use Magento\Framework\Serialize\SerializerInterface;
use Magento\Framework\View\Element\Template;
class Index extends \Magento\Framework\View\Element\Template {
private $cacheId = 'mySerializedData';
/**
* @var SerializerInterface
*/
private $serializer;
/**
* @param Template\Context $context
* @param SerializerInterface $serialized
* @param array $data
*/
public function __construct(
\Magento\Catalog\Block\Product\Context $context,
SerializerInterface $serializer,
array $data = []
) {
parent::__construct($context, $data);
$this->serializer = $serializer;
}
public function loadDataFromCache(){
$data = $this->_cache->load($this->cacheId);
$data = $this->serializer->unserialize($data);
return $data;
}
public function saveDataInsideCache(){
$this->insertData();
$data = $this->serializer->serialize($this->getData());
$this->_cache->save($data, $this->cacheId);
}
public function insertData(){
$data = [
'id' => 1,
'value' => 'Demo',
'email' => 'Amit.J.Tiwari@aureatelabs.com'
];
$this->setData($data);
}
protected function _prepareLayout()
{
return parent::_prepareLayout();
}
}
Step 5: Create a serializerdemo.phtml file inside the View/Frontend directory.
File Path: <root>/app/code/Aureatelabs/SerializerDemo/view/frontend/templates/serializerdemo.phtml
<?php
/**
* @var \Aureatelabs\SerializerDemo\Block\Index\Index $block
*/
$block->saveDataInsideCache();
$data = $block->loadDataFromCache();
?>
<h3>Unserialize Data</h3>
<p>Id: <?= $data['id'] ?></p>
<p>Value: <?= $data['value'] ?></p>
<p>Email Address: <?= $data['email'] ?></p>
Step 6: Please enabled and install the module using the below commands.
php bin/magento module:enable Aureatelabs_SerializerDemo
php bin/magento setup:upgrade
php bin/magento setup:di:compile
That’s it, we have successfully Implemented SerializerInterface in Magento 2. You can serialize and unserialize data by using the above solution but do remember this solution will only work with Magento 2.2 or higher.
Thank you.
Post a Comment
Got a question? Have a feedback? Please feel free to leave your ideas, opinions, and questions in the comments section of our post! ❤️