How to get customer order detail using GraphQl in Magento 2?
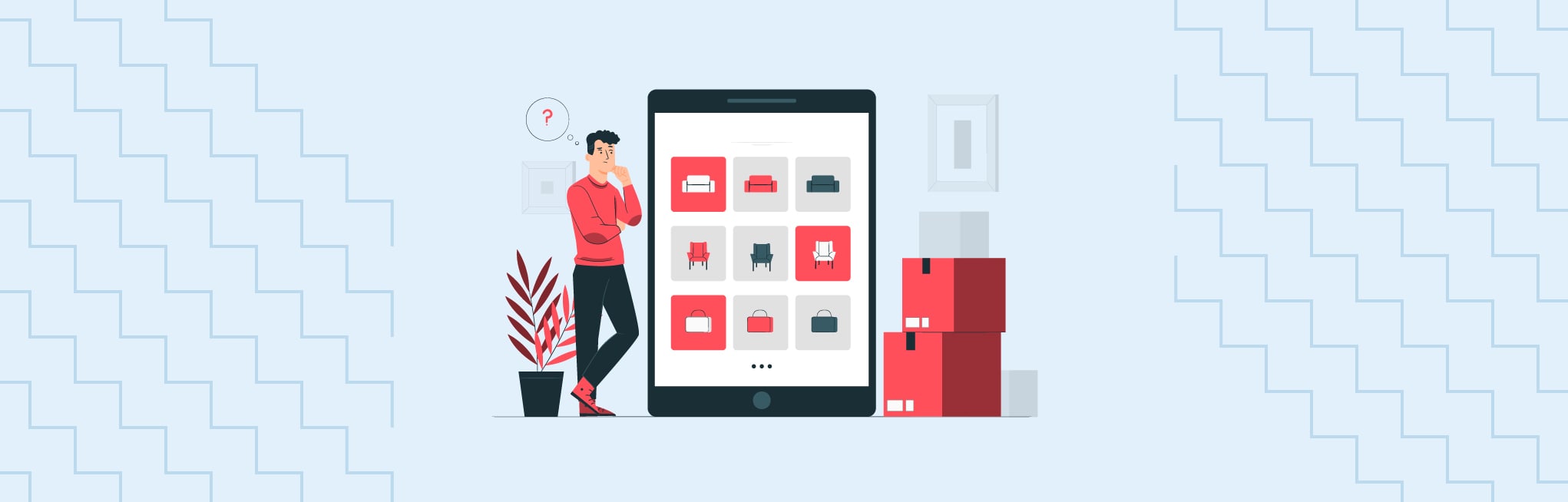
Here, in this blog, I will be guiding you on how to create a custom GraphQl query and get order details for specific customer id in Magento 2.
GraphQL is a data query language developed internally by Facebook in 2012
GraphQL is a new concept from Magento 2.3 version in Magento. Using Graphql we can get sales order data. There are many default core modules using GraphQl for getting data like, Product, CMS Page, and Block, Customer module uses Graphql for getting data of a specific entity. Magento Doesn’t use GraphQl for Sales Specific entity in core module.
Here we will be using AureateLabs as the Vendor name and CustomerOrder as the name of the module. You can change this according to your Vendor and Module name.
Follow the below steps to create a module with a custom GraphQl query.
Step 1: Register module
Create registration.php file, path app/code/AureateLabs/CustomerOrder/registration.php
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'AureateLabs_CustomerOrder',
__DIR__
);
Next create module.xml file, path app/code/AureateLabs/CustomerOrder/etc/module.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="AureateLabs_CustomerOrder">
<sequence>
<module name="Magento_Sales"/>
<module name="Magento_GraphQl"/>
</sequence>
</module>
</config>
Our Module depends on GraphQL and Sales Module so we have given the dependency on module.xml file for each module.
Step 2: Create schema.graphqls
Every GraphQl Module must contain a schema.graphqls file under the etc folder of a module.
schema.graphqls is the heart of any GraphQL Query implementation and describes what data can be queried. So You can define your query data basic skeleton under the schema.graphqls file.
Next create schema.graphqls file, path app/code/AureateLabs/CustomerOrder/etc/schema.graphqls
type Query {
customerPlacedOrder (
customer_id: Int @doc(description: "Id of the Customer for fetch all the placed order")
): SalesOrderCollection @resolver(class: "AureateLabs\\CustomerOrder\\Model\\Resolver\\CustomerOrder") @doc(description: "The Sales Order query returns information about customer all placed order")
}
type SalesOrderCollection @doc(description: "Sales order item information") {
allOrderRecords: [OrderRecord] @doc(description: "An array containing order information")
}
type OrderRecord @doc(description: "Sales Order graphql gather Data of specific order information") {
increment_id: String @doc(description: "Increment Id of Sales Order")
customer_name: String @doc(description: "Customername of Sales Order")
grand_total: String @doc(description: "Grand total of Sales Order")
created_at: String @doc(description: "Timestamp indicating when the order was placed")
shipping_method: String @doc(description: "Shipping method for order placed")
}
In the Schema file, We have passed customer_id for getting customer id as input. In SalesOrderCollection type for getting all the records as an array,
We have created an array for [OrderRecord]. In the type OrderRecord, we have to display all the fields which we required in the response to the query. You can add an extra field also here in the above schema.
Step 3: Create Resolver Model
Now Create a Resolver Model for our custom module. AureateLabs\\CustomerOrder\\Model\\Resolver\\CustomerOrder.php CustomerOrder Model class which is defined in schema.graphqls file at above. In this Model, resolve() method will simply return data of all the Orders of the specific customer
Next create CustomerOrder.php file, path app/code/AureateLabs\CustomerOrder\Model\Resolver\CustomerOrder.php
<?php
declare(strict_types=1);
namespace AureateLabs\CustomerOrder\Model\Resolver;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Framework\GraphQl\Config\Element\Field;
use Magento\Framework\GraphQl\Exception\GraphQlInputException;
use Magento\Framework\GraphQl\Exception\GraphQlNoSuchEntityException;
use Magento\Framework\GraphQl\Query\ResolverInterface;
use Magento\Framework\GraphQl\Schema\Type\ResolveInfo;
/**
* Order sales field resolver, used for GraphQL request processing
*/
class CustomerOrder implements ResolverInterface
{
public function __construct(
\Magento\Sales\Api\OrderRepositoryInterface $orderRepository,
\Magento\Framework\Api\SearchCriteriaBuilder $searchCriteriaBuilder,
\Magento\Framework\Pricing\PriceCurrencyInterface $priceCurrency,
\Magento\Directory\Model\Currency $currencyFormat
) {
$this->orderRepository = $orderRepository;
$this->searchCriteriaBuilder = $searchCriteriaBuilder;
$this->priceCurrency = $priceCurrency;
$this->currencyFormat =$currencyFormat;
}
/**
* Get current store currency symbol with price
* $price price value
* true includeContainer
* Precision value 2
*/
public function getCurrencyFormat($price)
{
return $this->getCurrencySymbol() . number_format((float)$price, \Magento\Framework\Pricing\PriceCurrencyInterface::DEFAULT_PRECISION);
}
/**
* Get current store CurrencySymbol
*/
public function getCurrencySymbol()
{
$symbol = $this->priceCurrency->getCurrencySymbol();
return $symbol;
}
/**
* @inheritdoc
*/
public function resolve(
Field $field,
$context,
ResolveInfo $info,
array $value = null,
array $args = null
) {
$customerId = $this->getCustomerId($args);
$salesData = $this->getSalesData($customerId);
return $salesData;
}
/**
* @param array $args
* @return int
* @throws GraphQlInputException
*/
private function getCustomerId(array $args): int
{
if (!isset($args['customer_id'])) {
throw new GraphQlInputException(__('"Customer id must be specified'));
}
return (int)$args['customer_id'];
}
/**
* @param int $customerId
* @return array
* @throws GraphQlNoSuchEntityException
*/
private function getSalesData(int $customerId): array
{
try {
/* filter for all customer orders */
$searchCriteria = $this->searchCriteriaBuilder->addFilter('customer_id', $customerId,'eq')->create();
$orders = $this->orderRepository->getList($searchCriteria);
$salesOrder = [];
foreach($orders as $order) {
$orderId = $order->getId();
$salesOrder['allOrderRecords'][$orderId]['increment_id'] = $order->getIncrementId();
$salesOrder['allOrderRecords'][$orderId]['customer_name'] = $order->getCustomerFirstname().' '.$order->getCustomerLastname();
$salesOrder['allOrderRecords'][$orderId]['grand_total'] = $this->getCurrencyFormat($order->getGrandTotal());
$salesOrder['allOrderRecords'][$orderId]['created_at'] = $order->getCreatedAt();
$salesOrder['allOrderRecords'][$orderId]['shipping_method'] = $order->getShippingMethod();
}
} catch (NoSuchEntityException $e) {
throw new GraphQlNoSuchEntityException(__($e->getMessage()), $e);
}
return $salesOrder;
}
}
We have set logic for getting all the orders from OrderRepositoryInterface. You need to set logic in the above file for display response.
Step 4: Install module and check
Now run the below command to install our module
php bin/magento setup:upgrade
php bin/magento cache:flush
You can check your GraphQL query response by installing chrome extension ChromeiQL or Altair GraphQL addon.
Open ChromeiQl and set endpoint {YOUR_SITE_FRONT_URL}/graphql here http://magento234.local is my local project url so my endpoint is http://magento234.local/graphql
In the Request Body, You need to pass the required data(field). I’m passing customer_id 1 for getting all the orders of a customer id 1.
Request Payload
{
customerPlacedOrder (customer_id: 1) {
allOrderRecords{
increment_id
customer_name
grand_total
created_at
shipping_method
}
}
}
Result: The result will be an all Order list of which customer ID is 1.
{
"data": {
"customerPlacedOrder": {
"allOrderRecords": [
{
"increment_id": "000000001",
"customer_name": "Veronica Costello",
"grand_total": "$36.39",
"created_at": "2020-04-24 06:44:58",
"shipping_method": "flatrate_flatrate"
},
{
"increment_id": "000000002",
"customer_name": "Veronica Costello",
"grand_total": "$39.64",
"created_at": "2020-04-24 06:45:09",
"shipping_method": "flatrate_flatrate"
},
{
"increment_id": "000000003",
"customer_name": "Veronica Costello",
"grand_total": "$162.38",
"created_at": "2020-05-24 05:39:26",
"shipping_method": "tablerate_bestway"
} ]
}
}
}
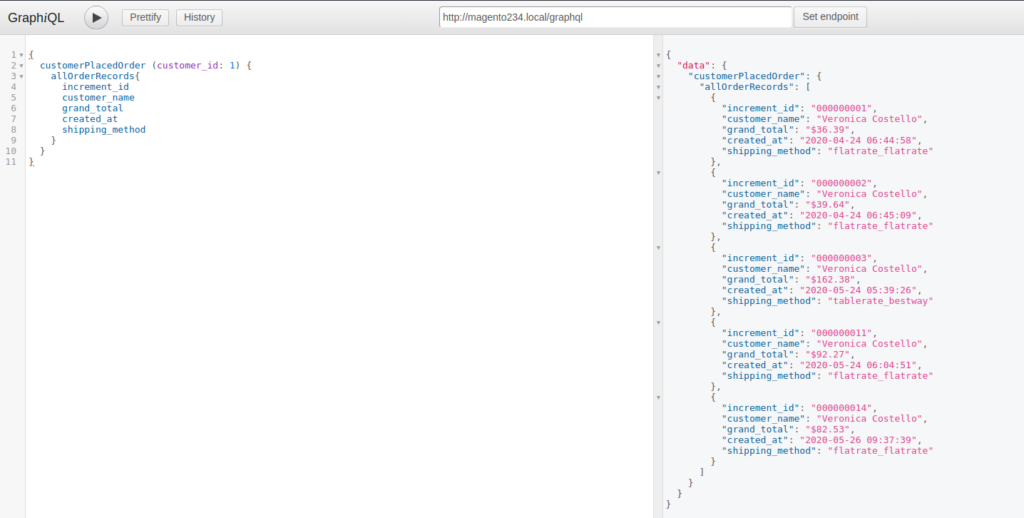
Reference screenshot: https://www.screencast.com/t/jl1YZtdWUE
Additional Information
Magento 2.3 and above have some inbuilt graphql you can test CMS Page GraphQl demo for access CMS Page data by GraphQL, Magneto 2.3 Comes with New Module called Magento_CmsGraphQl That module is responsible for CMS page GraphQl Query languages. You can get Page and Block’s Data using GraphQL.
Body for CMS Page request in GraphQL
{
cmsPage(
id: 2
) {
url_key
title
content
content_heading
page_layout
meta_title
meta_keywords
meta_description
}
}
For the above request body, a response would be like
{
"data": {
"cmsPage": {
"url_key": "home",
"title": "Home Page",
"content": "",
"content_heading": "Home Page",
"page_layout": "1column",
"meta_title": null,
"meta_keywords": "",
"meta_description": ""
}
}
}
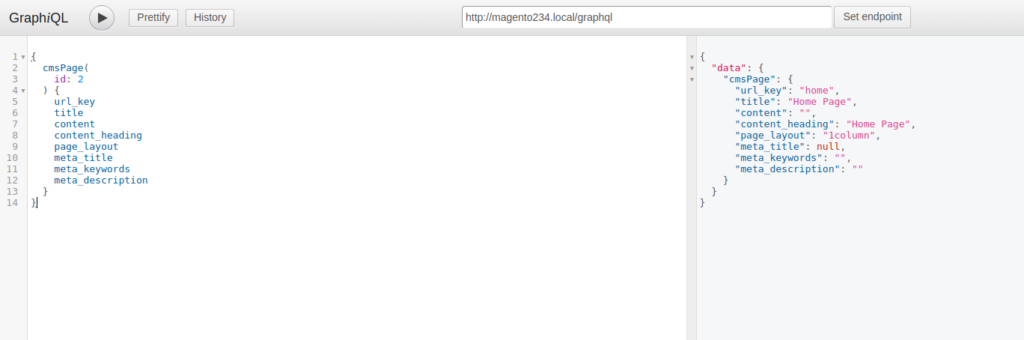
I hope you got better idea on how you can get customer order detail using GraphQl in Magento 2. If you have any question, please comment down below. I will be more than happy to reply you back.
Thanks for reading…
Post a Comment
Got a question? Have a feedback? Please feel free to leave your ideas, opinions, and questions in the comments section of our post! ❤️