In this post, I will be guiding you on how to use private content or sections while developing e-commerce store on Magento 2
Since private content is specific to individual users, it’s reasonable to handle it on the client side i.e. web browser.
A section is a piece of customer data grouped together. Each section is represented by the key that is used to access and manage data itself.
Magento loads sections by AJAX request to /customer/section/load/ and caches loaded data in the browser local storage under the key mage-cache-storage. Magento tracks when some section is changed and load updated section automatically.
Here we will be using Aureatelabs as the Vendor name and CustomSection as the name of the module. You can change this according to your Vendor and Module name.
Follow the below steps to define the custom section and use the data of the custom section in our custom phtml file.
Step 1: Define a custom section
We will define a custom section in the di.xml file by adding a new section into sections pool.
Create di.xml file in app/code/Aureatelabs/CustomSection/etc/frontend
directory.
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\Customer\CustomerData\SectionPoolInterface">
<arguments>
<argument name="sectionSourceMap" xsi:type="array">
<item name="custom_section" xsi:type="string">Aureatelabs\CustomSection\CustomerData\CustomSection</item>
</argument>
</arguments>
</type>
</config>
Next, create CustomSection.php file in app/code/Aureatelabs/CustomSection/CustomerData
directory.
<?php
namespace Aureatelabs\CustomSection\CustomerData;
use Magento\Customer\CustomerData\SectionSourceInterface;
class CustomSection implements SectionSourceInterface
{
public function getSectionData()
{
return [
'customdata' => "We are getting data from custom section",
];
}
}
In getSectionData()
method we will define our data that we want to store in section.
Step 2: Display custom section data in frontend
We will display our custom section data in the product view page. You can get section data in any Magento pages.
Next, we will create catalog_product_view.xml in app/code/Aureatelabs/CustomSection/view/frontend/layout
directory.
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceContainer name="product.info.main">
<block class="Magento\Catalog\Block\Product\View" name="aureatelabs_custom_section" after="-" template="Aureatelabs_CustomSection::custom_section.phtml" />
</referenceContainer>
</body>
</page>
Next, we will create a custom_section.phtml in app/code/Aureatelabs/CustomSection/view/frontend/templates
directory.
<div class="aureatelabs-custom-section"data-bind="scope: 'section'">
<p data-bind="text: customsection().customdata"></p>
</div>
<script type="text/x-magento-init">
{
"*": {
"Magento_Ui/js/core/app": {
"components": {
"section": {
"component": "Aureatelabs_CustomSection/js/section"
}
}
}
}
}
</script>
Next, We will create section.js in app/code/Aureatelabs/CustomSection/view/frontend/web/js
directory.
define([
'uiComponent',
'Magento_Customer/js/customer-data'
], function (Component, customerData) {
'use strict';
return Component.extend({
initialize: function () {
this._super();
this.customsection = customerData.get('custom_section');
}
});
});
Now run the below command and check on any product view page.
php bin/magento setup:upgrade
php bin/magento setup:di:compile
php bin/magento cache:flush
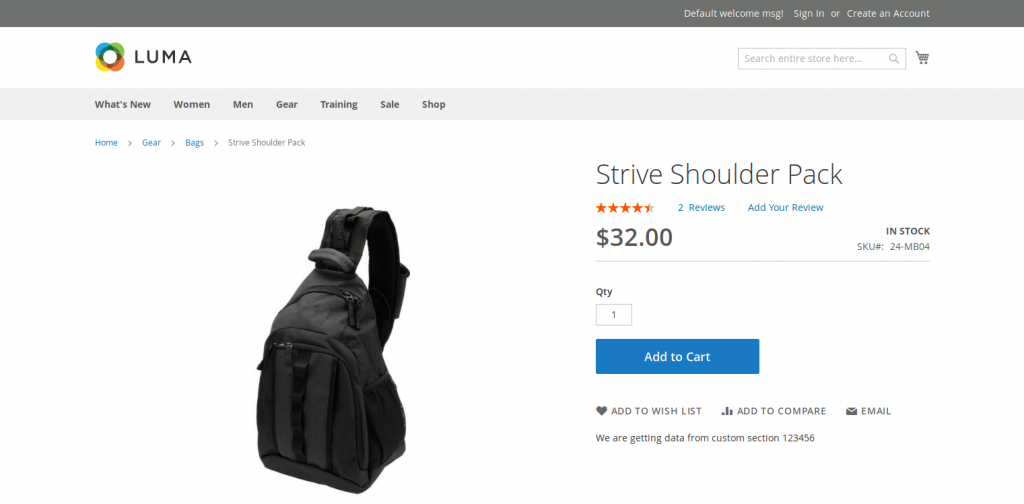
You can see the custom section data in the product view page.
You can also verify custom section is loaded or not by running below url
{store_url}/customer/section/load/?sections=custom_section
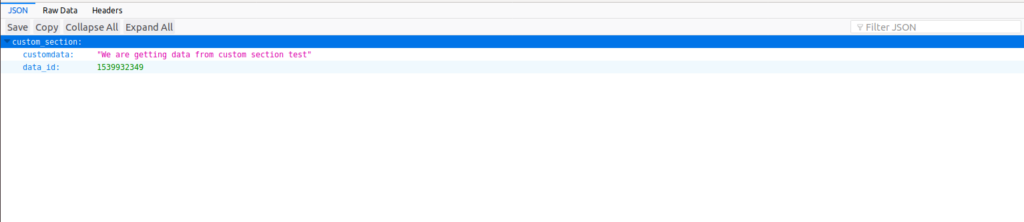
You can see custom section data in browser local storage.
In the section.js file, we have used Magento_Customer/js/customer-data as this Magento js library file is responsible for set section data and get section data. Section data gets updated when ajax call with the post, put, delete requests are made.
Additional Information
When caching is enabled the whole page content is cached so sections help us to dynamically manage the components of the page.
For example, the complete page is cached, Now when the customer logs in or logs out only the components get updated with the value from the section as you can see the customer name, cart, wish list are all managed by section.
All sections data are fetched in one single ajax call, hence the number of ajax requests are reduced to a huge number.
Magento assumes that section data is changed when a customer sends some state modification request (POST, PUT, DELETE). To minimize the load on the server, developers should specify which action (or request) updates which customer data section in etc/frontend/sections.xml
.
You can refer Magento/Catalog/etc/frontend/sections.xml
file.
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Customer:etc/sections.xsd">
<action name="catalog/product_compare/add">
<section name="compare-products"/>
</action>
<action name="catalog/product_compare/remove">
<section name="compare-products"/>
</action>
<action name="catalog/product_compare/clear">
<section name="compare-products"/>
</action>
<action name="customer/account/logout">
<section name="recently_viewed_product"/>
<section name="recently_compared_product"/>
</action>
</config>
In the section.xml file, they have defined update the compare-products section when one of these actions catalog/product_compare/add
, catalog/product_compare/remove
, catalog/product_compare/clear
are requested.
If the action name is * that means that section will be updated on each POST and PUT request. If the section tag is missed then all sections will be updated.
You can refer Magento/Theme/etc/frontend/sections.xml
file.
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Customer:etc/sections.xsd">
<action name="*">
<section name="messages"/>
</action>
</config>
In the section.xml file, they have defined update the messages section on each POST and PUT request.
If you want to reload your custom section data when the page refreshed then you have to reload your custom section data in your js file.
define([
'uiComponent',
'Magento_Customer/js/customer-data'
], function (Component, customerData) {
'use strict';
return Component.extend({
initialize: function () {
this._super();
customerData.reload('custom_section');
this.customsection = customerData.get('custom_section');
}
});
});
Here we have added customerData.reload('custom_section');
to reload section data whenever we refresh the page.
FAQs
Private content in Magento 2 is data accessible only to a particular user, based on the configurational changes made by them. This can be anything from purchase history to payment information. Magento 2 uses customer-data.js library to check if a customer is logged in, and then sends server requests to reload only a part of the data without refreshing the entire page cache.
To override layout XML in Magento 2, create a folder structure with the same name in themes as present in view/frontend. Then, change the etc/module.xml file and replace it by the new file created. You can do this by placing the file in app/design/frontend/[Vendor]/[Theme_Name]/Vendor_ModuleName/layout/some_layout.xml
The config.xml in Magento is located in the DOMAIN_HOME/config directory. The servers get their configuration data from these files. Each server has its own configuration file in the domain which can be accessed through <module_folder>/etc/adminhtml/system.xml. The config.xml files are used to set specific values for the configuration of the system.
To use section XML in Magento 2, create a new layout XML file in the app/code/[VendorName]/[ModuleName]/view/frontend/layout directory. Then, refresh the cache and view any product page to view section data. In Magento 2, sections define the layout configuration for different store areas, such as the header, footer, and content.
Related resources:
customerData.reload(‘custom_section’);
should be
customerData.reload([‘custom_section’]);