In modern web development, creating dynamic and functional URLs is a crucial aspect of building a seamless user experience. In today’s article, we’ll explore how to work with URLs in JavaScript within the context of the Hyva theme, commonly used with Magento.
We’ll cover obtaining the base URL, constructing custom URLs, handling parameters, and integrating these techniques into your Hyva theme.
Understanding Base URL in Hyva Theme
In Hyva theme, Magento 2 is often used as the backend, and it provides a window.BASE_URL global variable that holds the base URL of your Magento 2 store.
// Get the base URL in Hyva theme
const baseURL = window.BASE_URL;
console.log('Base URL:', baseURL);
We can perform this code in browsers developer console.
Please find the following screenshot.

Learn about the Hyvä Theme from scratch!
Building Custom URLs
You can use the base URL to build custom URLs by appending specific paths to it. Here’s an example.
// Build a custom URL using the base URL
const baseURL = window.BASE_URL;
const customPath = 'custom-path';
const customURL = baseURL + customPath;
console.log('Custom URL:', customURL);
// For the template literal version
console.log(`${BASE_URL}customPath`);
We can perform this code in browsers developer console.
Please find the following screenshot.
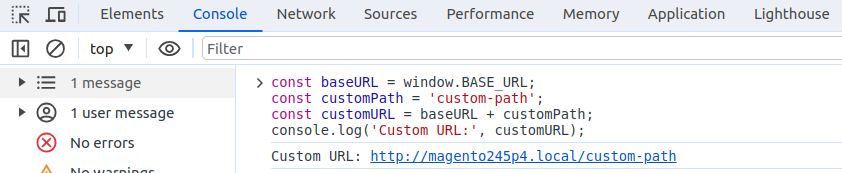
Handling URL Parameters
If your custom URL involves parameters, you can use the URLSearchParams API as below.
// Build a custom URL with parameters
const baseURL = window.BASE_URL;
const customPath = 'custom-path';
const customURL = baseURL + customPath;
const params = new URLSearchParams({
param1: 'value1',
param2: 'value2',
});
const customURLWithParams = baseURL + '/custom-path?' + params.toString();
console.log('Custom URL with Params:', customURLWithParams);
We can perform this code in browsers developer console.
Please find the following screenshot.
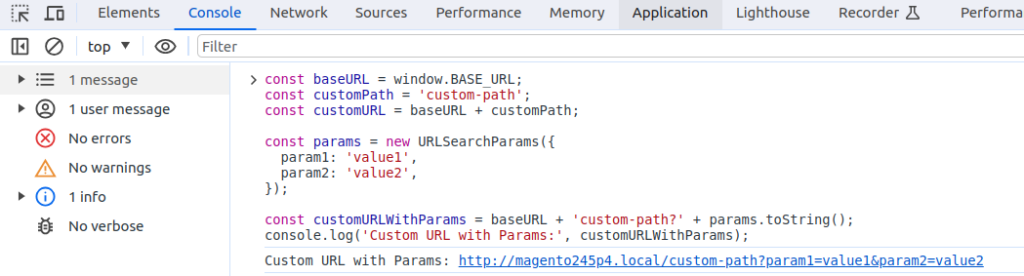
Displaying on the Frontend
Finally, you can display the URLs on your frontend or use them as needed in your JavaScript logic.
<!-- Displaying URLs on the frontend -->
<div>
<p>Base URL: <span id="base-url"></span></p>
<p>Custom URL: <span id="custom-url"></span></p>
<p>Custom URL with Params: <span id="custom-url-params"></span></p>
</div>
<script>
const baseURL = window.BASE_URL;
const customPath = 'custom-path';
const customURL = baseURL + customPath;
const params = new URLSearchParams({
param1: 'value1',
param2: 'value2',
});
const customURLWithParams = baseURL + '/custom-path?' + params.toString();
document.getElementById('base-url').textContent = baseURL;
document.getElementById('custom-url').textContent = customURL;
document.getElementById('custom-url-params').textContent = customURLWithParams;
</script>
We can perform this code in browsers developer console.
Please find the following screenshot.
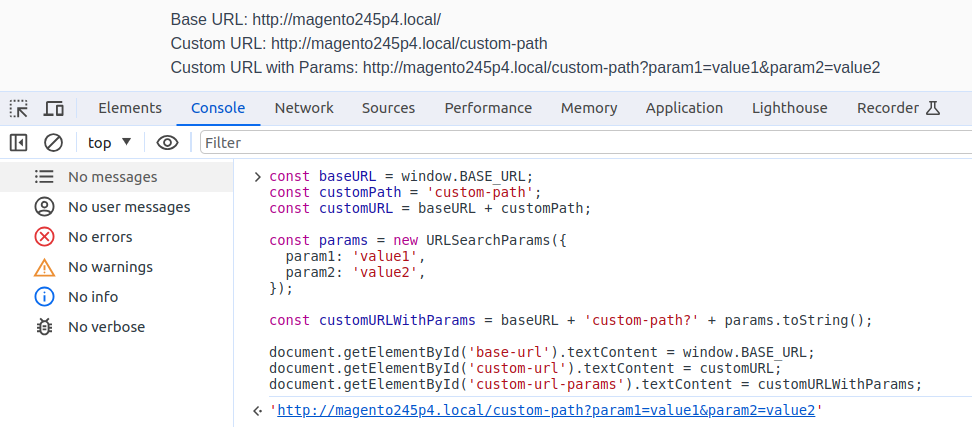
Integration with Hyva Theme
Make sure to integrate this JavaScript code into your Hyva theme appropriately. You may need to place it in the correct JavaScript file or customize your theme’s templates.
Remember to replace /custom-path, param1, and param2 with your actual paths and parameter names.
These steps should help you get started with obtaining the base URL and building custom URLs in the Hyva theme using JavaScript. Feel free to adapt the code to fit the specific requirements of your project.
Conclusion
Mastering the art of building URLs in JavaScript within the Hyva theme empowers developers to create dynamic and user-friendly web applications. Understanding the base URL, constructing custom URLs, handling parameters, and seamlessly integrating these techniques into your Hyva theme will contribute to a more efficient and engaging user experience.
Read more resources on Hyva themes:
- Check out the InstaBuild for Hyvä Themes: Ready-made Hyva theme templates for faster storefront development!
- Hyva Themes Development – For Online Merchants
- Hyvä Theme Benefits that your store needs
- 10 Best Hyva Compatibility Extension Providers For Magento 2 Stores
- Hyvä VS PWA: Where to Invest?
- How a Slow Magento Website Can Cost You Advertising Dollars
- Mobile Commerce: How Hyva Themes Boost Mobile Conversions
Post a Comment
Got a question? Have a feedback? Please feel free to leave your ideas, opinions, and questions in the comments section of our post! ❤️