In this post, I will be guiding you on:
- How Private section data is initialized?
- How can we get Private Section Data in Alpine.js components?
- How can we get Private Section Data in native JavaScript?
- Reloading / Invalidating Section Data on the Client side
- Reloading / Invalidating Section Data on the Server side
In the Hyva theme, the logic of private section data is much simplified than what we used in Default Luma Magento.
Since private content is specific to individual users, it’s reasonable to handle it on the client side i.e. web browser. A section data is a piece of customer data grouped together and each section is represented by the key.
Initialization of Private section data
Initialization of Private section data is triggered in the footer of every page. You can check the below code on how Section Data is triggered in the footer.
// this happens in the footer of every page
{
function dispatchPrivateContent(data) {
const privateContentEvent = new CustomEvent("private-content-loaded", {
detail: {
data: data
}
});
window.dispatchEvent(privateContentEvent);
}
function loadSectionData() {
// 1. load privateContent from localStorage.
//
// 2. determine if privateContent is valid.
// invalidation happens after 1 hour,
// or if the private_content_version cookie changed.
//
// 3. fetch privateContent from /customer/section/load if needed
//
// 4. dispatch privateContent event
dispatchPrivateContent(hyva.getDummyPrivateContent());
}
window.addEventListener("load", loadSectionData);
window.addEventListener("reload-customer-section-data", loadSectionData);
}
If there is no data present in LocalStorage, or one hour has passed or the private_content_version cookie is changed then fresh section data will be requested from /customer/section/load on when the next page loads and data stored in LocalStorage.
You can find the /customer/section/load request in the Browser’s Network tab on page load. You can also check the section data manually from the below URL.
{store_url}/customer/section/load/?sections=
Learn about the Hyvä Theme from scratch!
Get Private Section Data in Alpine.js components
We can receive the private section data in Alpine.js by listening to the “private-content-loaded” event on the window.
You can add the below code to get the private section data in the browser console.
<div x-data="" @private-content-loaded.window="console.log($event.detail.data)">
</div>
You will get the below response in the browser console when private section data is loaded.
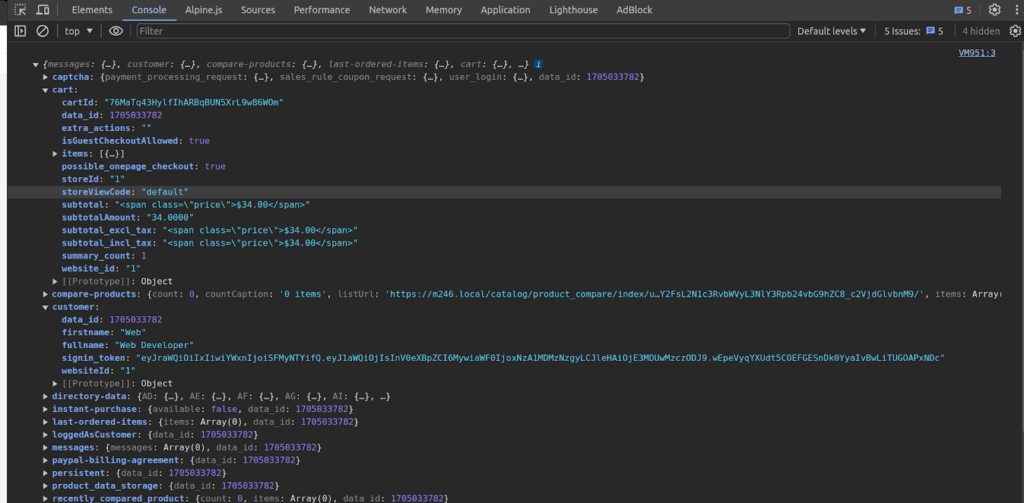
Image URL: https://www.screencast.com/t/txpJEAR46
As you can see when private section data is loaded, with the private-content-loaded event you will get all section data like customer, cart, wishlist, etc.
Note: In Hyvä it is not possible to subscribe to specific sections. All sections are always combined in one data object.
Let’s understand this with an example of getting customer data in Alpine js.
<script>
"use strict";
function initComponent() {
return {
customer: false,
getCustomerData(data) {
if (data.customer) {
this.customer = data.customer;
}
}
}
}
</script>
<div x-data="initComponent()"
@private-content-loaded.window="getCustomerData($event.detail.data)"
>
<template x-if="customer.fullname">
<div>Welcome, <span x-text="customer.fullname"></span></div>
</template>
<template x-if="!customer.fullname">
<div>Welcome Guest</div>
</template>
</div>
Output
- Guest user:
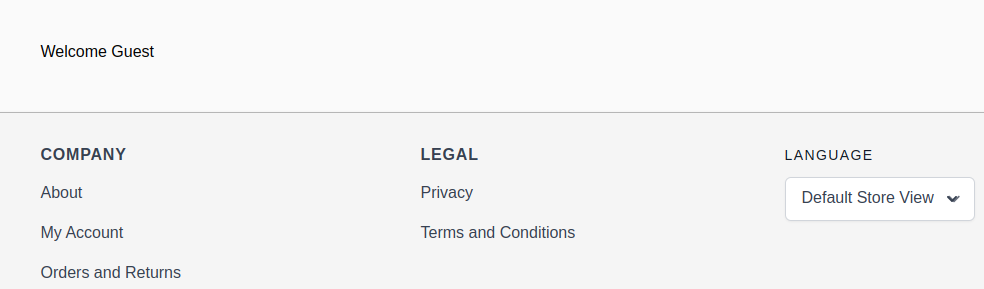
- Logged-in user:
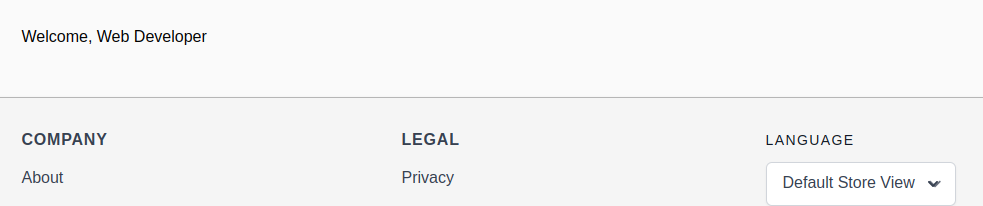
Get Private Section Data in native JavaScript
We can also get the section data using private-content-loaded events in native JavaScript.
<script>
"use strict";
function getCustomerData(event) {
const sectionData = event.detail.data;
if (sectionData.customer && sectionData.customer.fullname) {
console.log('Welcome, ' + sectionData.customer.fullname);
}
}
window.addEventListener("private-content-loaded", getCustomerData);
</script>
Output
You will get the below response in the browser console.
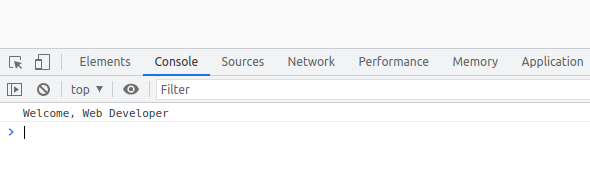
Reloading/Invalidating Section Data on the Client side
Reloading/Invalidating Section Data on the client side can be done with the dispatching “reload-customer-section-data” event.
window.dispatchEvent(new CustomEvent("reload-customer-section-data"));
Note:
- In contrast to Magento Luma themes, in Hyvä private content sections data are not invalidated/reloaded individually.
- The whole section data is only reloaded from the server if the current data is older than 1 hour, or the “private_content_version” cookie is changed.
- This can be either after a POST-request, or when the data is over 1 hour old.
When we dispatch the “reload-customer-section-data” event, it will cause the Section Data to be reloaded if it is expired or the “private_content_version” cookie is changed, then the private-content-loaded event will be triggered again.
If you want to force-reload the Section Data, we can do this by removing the “mage-cache-sessid cookie” before dispatching the “reload-customer-section-data” event.
// remove the mage-cache-sessid cookie
hyva.setCookie('mage-cache-sessid', '', -1, true);
// reload the section data
window.dispatchEvent(new CustomEvent("reload-customer-section-data"));
The private-content-loaded event will always be triggered after reloading the section data, regardless of whether it was requested from the server or read from local storage.
Reloading/Invalidating Section Data on the Server side
If you want to refresh the Section Data from the Server side(Backend) then the mage-cache-sessid cookie or the private_content_version cookie needs to be deleted.
Magento has done this to refresh the Section Data on any frontend or GraphQL POST request (but not for the REST API). You can check the “process” function in the Magento\Framework\App\PageCache\Version class.
public function process()
{
if ($this->request->isPost()) {
$publicCookieMetadata = $this->cookieMetadataFactory->createPublicCookieMetadata()
->setDuration(self::COOKIE_PERIOD)
->setPath('/')
->setSecure($this->request->isSecure())
->setHttpOnly(false)
->setSameSite('Lax');
$this->cookieManager->setPublicCookie(self::COOKIE_NAME, $this->generateValue(), $publicCookieMetadata);
}
}
You can do the same thing in custom PHP code during any POST request, inject class Magento\Framework\App\PageCache\Version, and call $version->process().
You can force the refresh in a GET request, and copy the code from Version::process. Keep in mind that GET request responses are usually cached in the FPC.
Read more resources on Hyva themes:
- Check out the InstaBuild for Hyvä Themes: Ready-made Hyva theme templates for faster storefront development!
- Hyva Themes Development – For Online Merchants
- Hyvä Theme Benefits that your store needs
- 10 Best Hyva Compatibility Extension Providers For Magento 2 Stores
- Hyvä VS PWA: Where to Invest?
- How a Slow Magento Website Can Cost You Advertising Dollars
- Mobile Commerce: How Hyva Themes Boost Mobile Conversions
Post a Comment
Got a question? Have a feedback? Please feel free to leave your ideas, opinions, and questions in the comments section of our post! ❤️